AngularJS: The Ultimate Guide for Developers
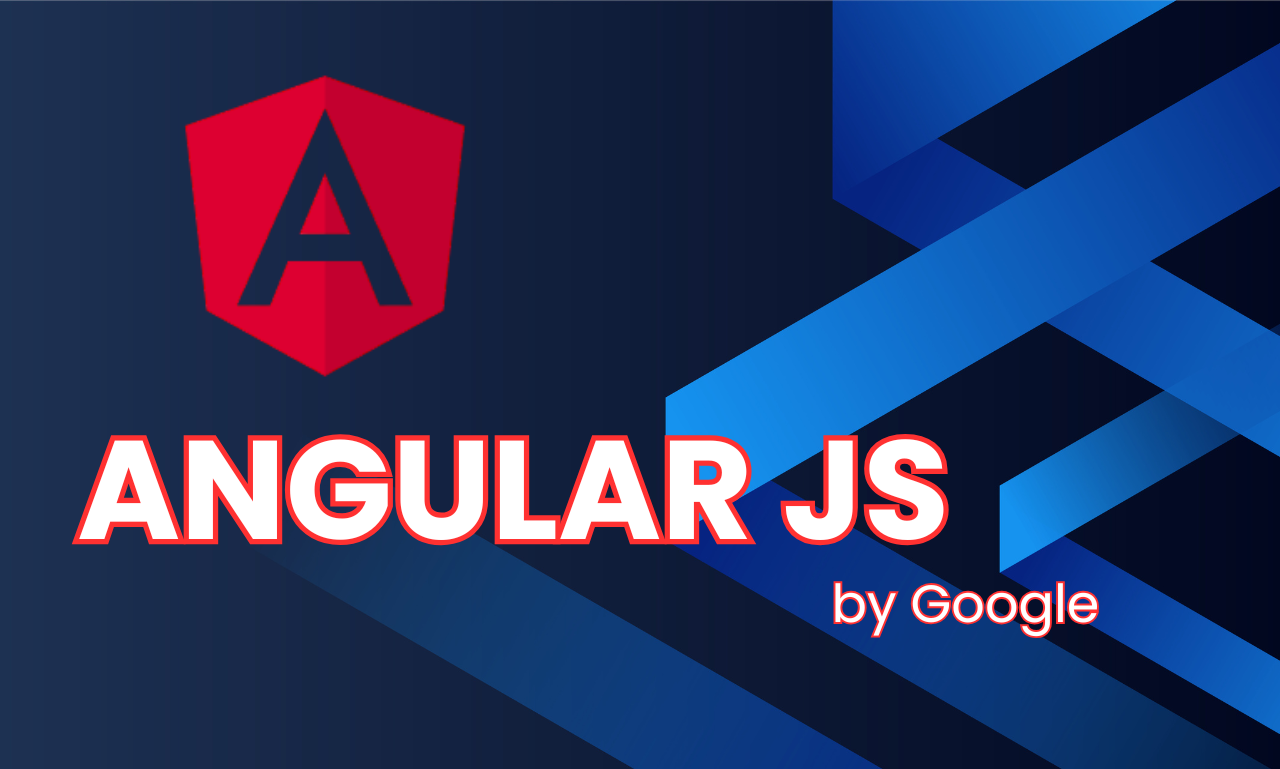
AngularJS has left an indelible mark on the world of web development. Whether you’re building single-page applications (SPAs) or creating dynamic, feature-rich websites, AngularJS provides the tools you need to succeed. This guide takes you through everything you need to know about AngularJS, from its history and key features to practical tips for implementation, advanced techniques, and its role in the future of web development. Let’s explore why AngularJS deserves a spot in every developer’s toolkit.
Introduction
AngularJS, developed by Google in 2010, revolutionized how we build and maintain web applications. Unlike traditional static HTML, AngularJS introduces a framework that allows dynamic views by coupling HTML with JavaScript functionality. It uses client-side MVC (Model-View-Controller) architecture, making it an essential tool for creating fast, scalable, and maintainable web applications.
Since its inception, AngularJS has garnered immense popularity among developers for its ability to handle complex web applications with ease. While newer frameworks like React and Vue.js have gained traction, AngularJS remains a strong choice for many developers and businesses.
Key Features and Benefits of AngularJS
AngularJS isn’t just another JavaScript framework. It’s a robust platform packed with features designed to simplify web development.
Key Features
- Data Binding: Two-way data binding ensures real-time synchronization between the model and the view. This eliminates the need for manual DOM manipulations.
- Dependency Injection: Manages the lifecycle of components and resolves dependencies, leading to cleaner code and improved testability.
- Directives: AngularJS extends HTML functionality with directives, enabling developers to create custom and reusable HTML components.
- MVVM Architecture: Simplifies organizing code by separating the Model, View, and ViewModel, improving maintainability and scalability.
- Testing: Comes with built-in unit testing and end-to-end testing capabilities, ensuring application reliability.
Benefits for Developers and Businesses
- Efficiency: AngularJS reduces development time by automating routine tasks like DOM manipulation and data synchronization.
- Flexibility: Its modular approach allows developers to reuse components and scale applications efficiently.
- Community Support: Backed by Google and a vast developer community, AngularJS offers regular updates, plugins, and extensive documentation.
- Cost Savings: By streamlining the development process, AngularJS helps businesses save resources and time.
Getting Started with AngularJS
For those new to AngularJS, setting up can be quick and straightforward. Here’s a step-by-step guide to get you started.
Step 1: Install AngularJS
- Download AngularJS from the official website. Alternatively, include the AngularJS CDN in your project’s `<script>` tag.
- Link AngularJS to your HTML file with:
```html
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.0/angular.min.js"></script>
```
Step 2: Add the ng-app Directive
Integrate AngularJS into your HTML by adding the `ng-app` directive, which initializes your AngularJS application.
```html
<html ng-app="myApp">
```
Step 3: Create a Module and Controller
1. Define a module in JavaScript using `angular.module`.
```javascript
var app = angular.module('myApp', []);
```
2. Add a controller to establish logic.
```javascript
app.controller('myCtrl', function($scope) {
$scope.message = "Welcome to AngularJS!";
});
```
Step 4: Bind Data to Your View
Easily link your application logic to the view using AngularJS’s `{{ }}` syntax.
```html
<div ng-controller="myCtrl">
{{ message }}
</div>
```
You’re now ready to build your first AngularJS application!
Advanced Techniques for AngularJS
Custom Directives
Create reusable components with custom directives to enhance your application’s structure.
```javascript
app.directive('customDirective', function() {return {
template: "<h1>This is a custom directive!</h1>"
};
});
```
Filters
Use filters to format data dynamically, such as transforming dates, currency, or uppercase strings.
```html
{{ amount | currency }}
```
Modular Development
Split your application into multiple modules for better organization and module-specific functionality.
```javascript
var mainApp = angular.module('mainApp', []);
var adminApp = angular.module('adminApp', []);
```
Optimize Performance
- Use `$watch` sparingly to minimize performance overhead.
- Reduce DOM manipulations by using `track by`.
- Leverage lazy loading for large applications.
AngularJS in Real-World Applications
AngularJS has powered some impressive web applications across industries. Notable examples include:
- Google uses AngularJS for its projects, reflecting support from the technology’s creator.
- YouTube PS3 Application relies on AngularJS for flexible and smooth user experiences.
- Weather.com employs AngularJS for scalable and visually engaging web applications.
AngularJS’s versatility has made it a staple for creating dashboards, single-page applications, and high-performance web solutions.
Comparison: AngularJS vs React
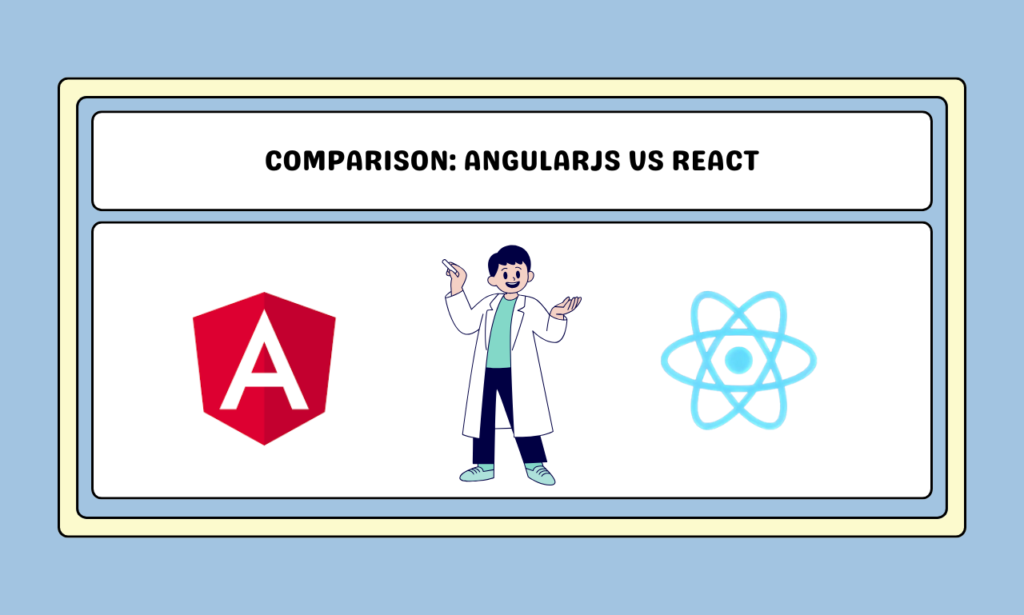
Feature | AngularJS (1.x) | React |
Release Year | 2010 | 2013 |
Developed By | Facebook (Meta) | |
Architecture | MVC (Model-View-Controller) | Component-based (Virtual DOM) |
Language | JavaScript | JavaScript (with JSX) |
Performance | Slower due to two-way data binding and digest cycle | Faster with Virtual DOM |
Data Binding | Two-way data binding | One-way data binding |
State Management | $scope and $rootScope | React’s useState and external libraries (Redux, Context API) |
DOM Manipulation | Direct DOM manipulation with watchers | Virtual DOM for efficient rendering |
Componentization | Uses directives and controllers | Fully component-based |
Mobile Support | Not optimized for mobile | React Native for cross-platform mobile apps |
Routing | Uses ngRoute module | Uses react-router for navigation |
SEO Optimization | Difficult, requires additional tools | Better SEO with server-side rendering (Next.js) |
Testing Support | Built-in but limited | Jest and React Testing Library |
Learning Curve | Steep due to its complex structure | Easier with component-based architecture |
Backward Compatibility | Not compatible with Angular 2+ | Backward compatible across versions |
Community Support | Large but declining | Large and rapidly growing |
Future Updates | No new updates (LTS ended in 2022) | Regular updates and strong ecosystem |
Use Cases | Legacy applications, SPAs | Modern web apps, interactive UIs, SPAs |
The Future of AngularJS
Google officially transitioned AngularJS to long-term support mode in 2021, encouraging developers to migrate to Angular (its successor). However, AngularJS’s simplicity, proven capabilities, and vast community support continue to make it relevant for specific use cases.
For businesses maintaining legacy systems, AngularJS remains a go-to option. Future updates may be limited compared to newer frameworks, but its rich ecosystem ensures it will remain a valuable tool.
Tips for Enhancing SEO with AngularJS
One common challenge in AngularJS applications is ensuring they are search engine-friendly. Here are some tips to optimize them.
- Enable Server-Side Rendering (SSR): Use tools like `Prerender.io` to serve static HTML to search engines.
- Dynamic Metadata: Use `$location` service to dynamically set titles and meta descriptions.
- Optimize Content Delivery: Minimize loading times by compressing assets and using Content Delivery Networks (CDNs).
- Progressive Enhancement: Ensure core functionality works even without JavaScript enabled.
By implementing these strategies, developers can make their AngularJS applications more visible to search engines.
Difference between AngularJS and Angular
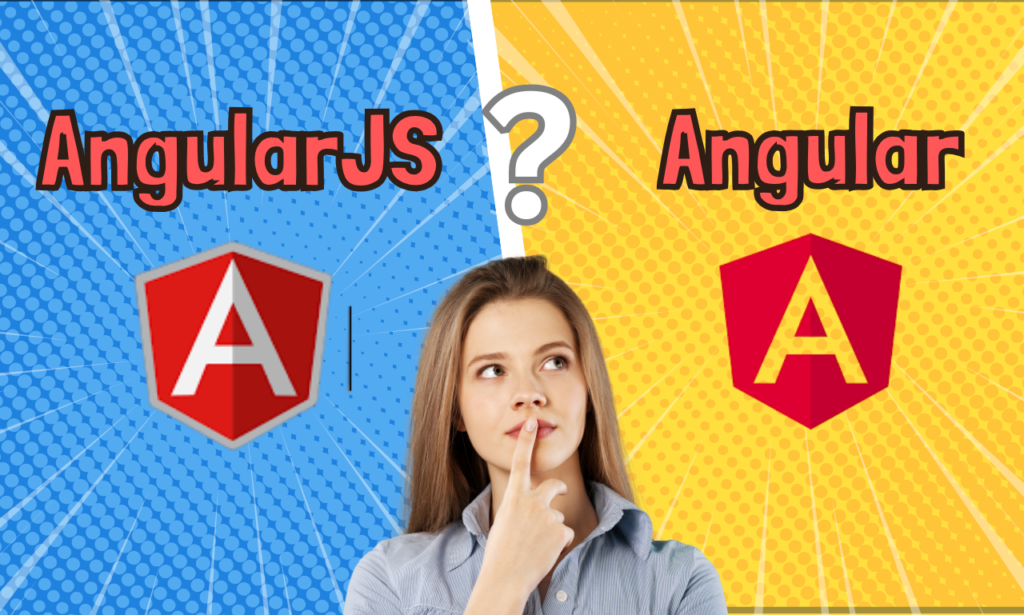
Feature | AngularJS (1.x) | Angular (2+) |
Release Year | 2010 | 2016 |
Developed By | ||
Architecture | MVC (Model-View-Controller) | Component-based architecture |
Language | JavaScript | TypeScript |
Performance | Slower due to two-way data binding and digest cycle | Faster with improved change detection (Zone.js) |
Data Binding | Two-way data binding | Two-way and one-way data binding |
Dependency Injection | Limited DI support | Advanced DI system |
Directives | Uses built-in directives like ng-model, ng-bind | Uses custom directives with @Directive decorator |
Mobile Support | Not optimized for mobile | Fully mobile-friendly and responsive |
Modularity | Less modular, relies on controllers and $scope | Highly modular, uses components and modules |
SEO Optimization | Difficult, requires additional tools | Improved SEO capabilities with Angular Universal |
Routing | Uses ngRoute module | Uses Angular Router with more features |
Testing Support | Built-in testing tools, but limited | Stronger testing tools with Jasmine and Karma |
Backward Compatibility | Not compatible with Angular 2+ | Not backward compatible with AngularJS |
Community Support | Large but declining due to newer alternatives | Active and growing community support |
Future Updates | No new updates (LTS ended in 2022) | Regular updates and long-term support |
Use Cases | Legacy applications, SPAs | Modern web apps, enterprise apps, PWAs |
Unlock the Potential of AngularJS Today
AngularJS remains a powerful tool in the web developer’s arsenal. From rapidly creating SPAs to delivering dynamic web content, AngularJS offers features that simplify and enhance the development process. Whether you’re a seasoned developer or stepping into the web development world, AngularJS’s versatility and ease of use make it worth exploring.
Start experimenting with AngularJS today and keep an eye on related trends for modern frameworks like Angular and React. With its enduring relevance and community support, AngularJS is still a fantastic choice for developers in 2024.
Feel inspired? Take your skills further by exploring more advanced AngularJS applications, or broaden your horizons with its successor, Angular.