Exploring Pure JavaScript: The Foundation of Modern Web Development
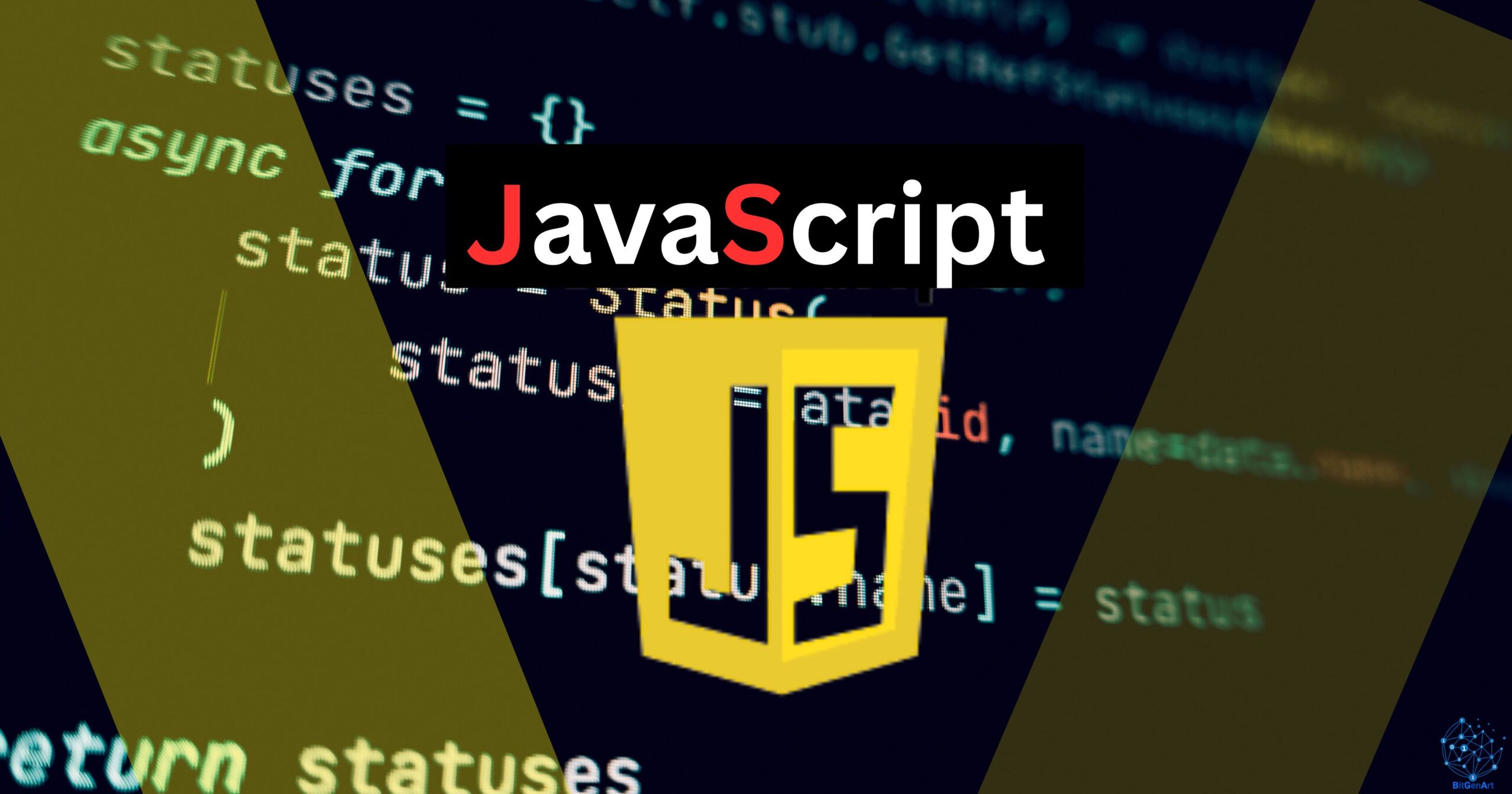
JavaScript is the backbone of modern web development. It brings interactivity and dynamic elements to websites, allowing developers to create responsive forms, interactive maps, real-time updates, and much more.
But what is Pure JavaScript? Also called “Vanilla JavaScript,” it refers to using JavaScript in its original form without relying on frameworks or libraries like React, Vue.js, or Angular. Understanding Vanilla JavaScript is essential for any developer who wants to have full control over their code and build applications from scratch.
In this article, we will explore what JavaScript is, its history, how it became a powerful programming language, and why learning Pure JavaScript is important. Let’s get started!
What is JavaScript?
JavaScript is a high-level, lightweight, and interpreted programming language. It is mainly used to add interactivity to web pages. While HTML structures a webpage and CSS styles it, JavaScript makes it dynamic and responsive.
Some common uses of JavaScript include:
- Handling user interactions like clicks, form submissions, and mouse movements.
- Fetching and displaying real-time data without requiring a page refresh.
- Creating animations and interactive elements like sliders and pop-ups.
- Developing web applications, browser-based games, and even mobile apps.
JavaScript is essential for web developers because it runs directly in the browser, eliminating the need for additional plugins or installations. It is also supported by all modern web browsers, making it a universal language for web development.
Key Features of Pure JavaScript
Using JavaScript without external frameworks or libraries has several advantages. Here are some key features of Pure JavaScript:
1.Client-Side Execution
JavaScript runs directly in the user’s web browser, reducing the load on the server and making web pages more efficient.
2.Dynamic Typing
JavaScript does not require explicit type declarations. This means variables can hold different types of values during runtime, making development more flexible.
3.Event-Driven Programming
JavaScript responds to user actions, such as clicks and keypresses, allowing developers to create highly interactive experiences.
4.Cross-Browser Compatibility
Modern JavaScript works on all major browsers, including Chrome, Firefox, Safari, Edge, and Opera, without requiring additional plugins.
5.Prototype-Based Inheritance
Unlike many object-oriented languages that use class-based inheritance, JavaScript uses prototypes, making it more flexible and powerful.
The History of JavaScript
JavaScript was created in 1995 by Brendan Eich while working at Netscape Communications Corporation. The goal was to make web pages more interactive and engaging. Initially, it was called “Mocha,” then renamed “LiveScript,” and finally “JavaScript.”
Key Milestones in JavaScript History:
- 1995: Brendan Eich developed JavaScript in just 10 days while working at Netscape.
- 1996: Microsoft introduced JScript, a variant of JavaScript, to work on Internet Explorer.
- 1997: JavaScript was standardized as ECMAScript (ES) by ECMA International.
- 2009: Node.js was released, allowing JavaScript to run on servers.
- 2015: ECMAScript 6 (ES6) introduced modern features like let and const, arrow functions, promises, and template literals.
- Present Day: JavaScript continues to evolve with annual updates, making it one of the most powerful and widely used languages.
Why is it Called JavaScript?
Many people mistakenly believe JavaScript is related to Java. In reality, the name was chosen for marketing purposes because Java was a popular language at the time. Despite their similar names, JavaScript and Java are entirely different languages.
How JavaScript Changed Web Development
Before JavaScript, websites were mostly static, displaying only text and images. Developers had to rely on backend languages like PHP or Perl to create dynamic elements. JavaScript changed this by allowing real-time interactions directly in the browser.
Some of the ways JavaScript revolutionized web development include:
- Making Web Pages Interactive: JavaScript enables features like animations, image sliders, and dropdown menus.
- Enhancing User Experience: Users can interact with web applications without constant page reloads.
- Enabling Frontend Frameworks: JavaScript is the foundation of frameworks like React, Angular, and Vue.js.
- Supporting Server-Side Development: With Node.js, JavaScript can now handle backend processes.
Where JavaScript is Used Today
JavaScript is not limited to web browsers. It is now used in various fields, including:
1.Web Development
JavaScript is essential for front-end development, making websites dynamic and responsive.
2.Web APIs
JavaScript connects front-end applications to backend services using APIs. It enables real-time data fetching, such as live sports scores and weather updates.
3.Game Development
JavaScript powers browser-based games using HTML5 and WebGL.
4.Mobile App Development
With frameworks like React Native and Ionic, JavaScript can be used to develop mobile applications for Android and iOS.
5.Server-Side Development
Node.js allows JavaScript to run on servers, making it possible to build full-stack applications using a single language.
6.Internet of Things (IoT)
JavaScript can control smart devices, sensors, and automation systems through lightweight frameworks.
Why Learn Pure JavaScript?
Many developers jump straight into frameworks without learning the core language. However, mastering Pure JavaScript has several benefits:
1.Understanding the Basics
Learning Pure JavaScript gives you a strong foundation, making it easier to work with frameworks like React, Angular, or Vue.
2.No Dependency on Updates
Frameworks change frequently, but JavaScript itself evolves gradually. Knowing the core language ensures you can adapt to new technologies.
3.Better Debugging and Problem-Solving
When you understand JavaScript deeply, you can troubleshoot and optimize your code more effectively.
4.Versatility
JavaScript is used in web, mobile, and server-side development, opening up numerous career opportunities.
Example of Pure JavaScript in Action
Here’s a simple example of how JavaScript can dynamically change text on a webpage.
HTML:(index.html)
<!DOCTYPE html> <html> <body> <p id="text">Hello, World!</p> <button onclick="changeText()">Click Me</button> <script src="script.js"></script> </body> </html>
JavaScript (script.js):
function changeText() { document.getElementById("text").innerHTML = "You clicked the button!"; }
This small script demonstrates how JavaScript interacts with HTML elements without any external libraries or frameworks.
Final Thoughts
From its early days at Netscape to its current role in full-stack development, JavaScript has become an essential tool for programmers worldwide. Learning Pure JavaScript gives you complete control over your code and allows you to build efficient and scalable applications.
Frameworks and libraries come and go, but a solid understanding of Vanilla JavaScript will always be valuable. By mastering the fundamentals, you can develop high-quality applications, troubleshoot issues effectively, and stay ahead in the fast-changing world of web development.
So, whether you’re a beginner or an experienced developer, investing time in Pure JavaScript is a decision that will benefit your programming journey for years to come. Happy coding!