Understanding dotenv: A Guide to Managing Environment Variables in Node.js
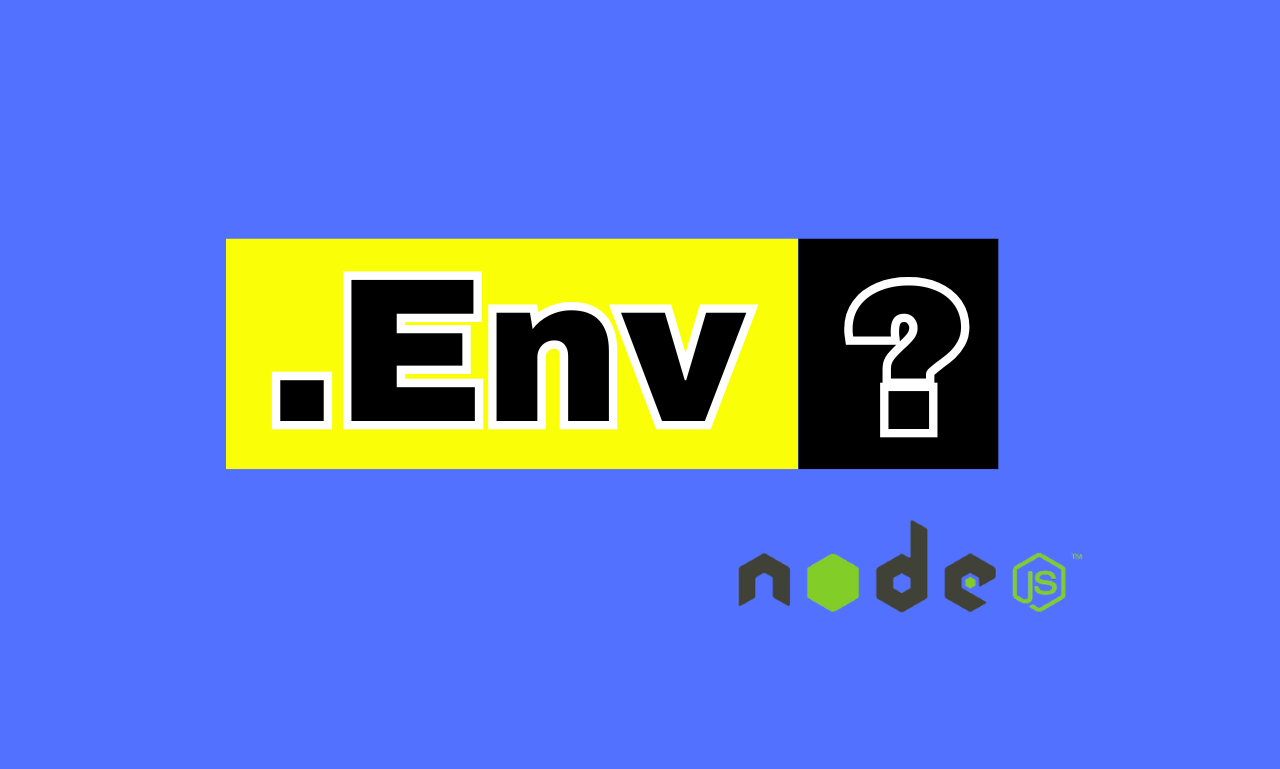
Introduction
In modern web development, managing environment variables securely is crucial. The dotenv package is a widely used Node.js module that allows developers to load environment variables from a .env
file into process.env
. This helps keep sensitive configuration details separate from the codebase, following the Twelve-Factor App methodology.
What is dotenv?
Dotenv is a zero-dependency package that simplifies handling environment variables in Node.js applications. It enables developers to define variables in a .env
file and access them within the application without exposing them in the source code.
Who Created Dotenv and When?
Dotenv was created by Scott M. Likens as a lightweight and efficient way to manage environment variables in applications. It has since become an essential tool for developers working with Node.js and JavaScript-based applications.
Dotenv was first released in 2013 as an open-source package. Over time, it has gained widespread adoption among developers due to its simplicity and effectiveness.
Why Use Dotenv in Node.js?
Using dotenv provides multiple benefits, including:
- Security: Prevents sensitive data from being exposed in public repositories.
- Ease of Use: Loads environment variables effortlessly into process.env.
- Portability: Allows developers to maintain different configurations for development, testing, and production.
- Cleaner Code: Keeps configuration values separate from the main codebase.
- Collaboration: Ensures multiple developers can work on a project without sharing credentials.
Use Cases of Dotenv
Dotenv is widely used in various scenarios, such as:
- Database Configurations: Managing credentials for MongoDB, PostgreSQL, or MySQL.
- API Key Management: Storing API keys for services like Stripe, OpenAI, or Google Maps.
- Authentication Secrets: Safeguarding JWT secret keys and OAuth credentials.
- Cloud Service Credentials: Managing AWS, Firebase, or Azure configurations.
- Feature Flagging: Enabling or disabling features based on environment settings
Installation
You can install dotenv using npm or yarn:
# Using npm (recommended)
npm install dotenv --save
# Using yarn
yarn add dotenv
How to Use dotenv
To use dotenv, create a .env
file in your project’s root directory and add key-value pairs representing environment variables:
S3_BUCKET="your_s3_bucket"
SECRET_KEY="your_secret_key_here"
Then, in your Node.js application, load the environment variables as early as possible:
// CommonJS
require('dotenv').config();
// ES6
import 'dotenv/config';
Now, you can access these variables using process.env
:
console.log(process.env.S3_BUCKET); // Outputs: your_s3_bucket
1. Multiline Values
If your environment variables require multiline values, such as private keys, you can define them like this:
PRIVATE_KEY="----BEGIN RSA PRIVATE KEY----\n...\n----END RSA PRIVATE KEY----"
2. Adding Comments
You can include comments in the .env
file for better readability:
# This is a comment
SECRET_KEY="your_secret_key" # Inline comment
3. Parsing Environment Variables
Dotenv provides a built-in parsing function:
const dotenv = require('dotenv');
const config = dotenv.parse(Buffer.from('API_KEY=12345'));
console.log(config); // { API_KEY: '12345' }
4. Ignore .env in Git
To prevent accidental exposure of sensitive information, add .env to your .gitignore file:
# .gitignore
.env
Advanced Usage
1. Preloading dotenv
Instead of manually requiring dotenv in your code, you can preload it using the -r
flag:
node -r dotenv/config app.js
This can also accept additional configurations:
node -r dotenv/config app.js
dotenv_config_path=.custom.env dotenv_config_debug=true
2. Managing Multiple Environments
For applications that require different environment configurations (e.g., development, testing, production), you can use multiple .env
files:
# Define different environment files
.env.development
.env.production
.env.testing
You can then specify which environment file to use when running the application:
DOTENV_CONFIG_PATH=.env.production node app.js
3. Deploying Securely
When deploying applications, avoid committing .env
files to version control. Instead, use tools like dotenvx to encrypt environment variables and manage them securely across different environments.
# Encrypt environment variables
dotenvx set API_KEY myapikey --encrypt -f .env.production
To use the encrypted file in production:
DOTENV_PRIVATE_KEY_PRODUCTION="your_private_key" dotenvx run -- node app.js
Rules for Using Dotenv
- Never commit your .env file to version control (e.g., GitHub).
- Use a .env.example file to share environment variable names without revealing sensitive data.
- Always load dotenv at the beginning of your application before accessing process.env.
- Validate environment variables to ensure required values are set before running the application.
- Use different .env files for different environments (e.g., .env.development, .env.production).
- Avoid hardcoding environment variable values inside your code.
- Ensure dotenv is installed as a dependency and not as a devDependency if required in production.
- Restart your application after changing .env file values for changes to take effect.
- Avoid exposing environment variables in frontend applications to prevent security risks.
- Use process managers like PM2 to set environment variables in production instead of dotenv.
Pros and Cons of Using Dotenv
Pros:
- Security: Keeps sensitive data out of the codebase.
- Easy to Implement: Requires minimal setup.
- Flexible: Works across different environments.
- Lightweight: Adds minimal overhead to the application.
Cons:
- Not Suitable for Production: Should not be used in production environments; use environment variables set in the OS instead.
- Performance Overhead: Parsing a .env file adds a minor performance cost.
- No Encryption: Dotenv does not encrypt environment variables; additional security measures may be needed.
Comparing Dotenv with Other Environment Variable Managers
Dotenv vs. Config.js
Feature | Dotenv | Config.js |
Ease of Use | Very easy | Requires additional setup |
Security | Basic | More advanced (supports encryption) |
Performance | Fast | Slightly slower due to added features |
Best For | Small to medium apps | Large applications |
Dotenv vs. Environment Variables in the OS
Feature | Dotenv | OS Variables |
Setup | Quick | Requires manual configuration |
Portability | High | Low |
Security | Low | High |
Best For | Development & Testing | Production |
Best Practices for Using Dotenv
- Never commit .env files to version control.
- Use .env.example to share environment variable names without revealing sensitive values.
- Validate environment variables to ensure required values are set before running the application.
- Use process managers like PM2 to set environment variables in production instead of dotenv.
- Avoid exposing environment variables in front-end applications as they can be accessed by users.
Final thought
Dotenv simplifies environment variable management, making it easier to keep sensitive information secure while maintaining flexibility in different deployment environments. By following best practices like preloading, multiple environment management, and secure deployment, developers can ensure a more maintainable and secure Node.js application.
Would you like more insights on how dotenv integrates with frameworks like Express or React? Let me know in the comments!