Web3.js: A Comprehensive Guide
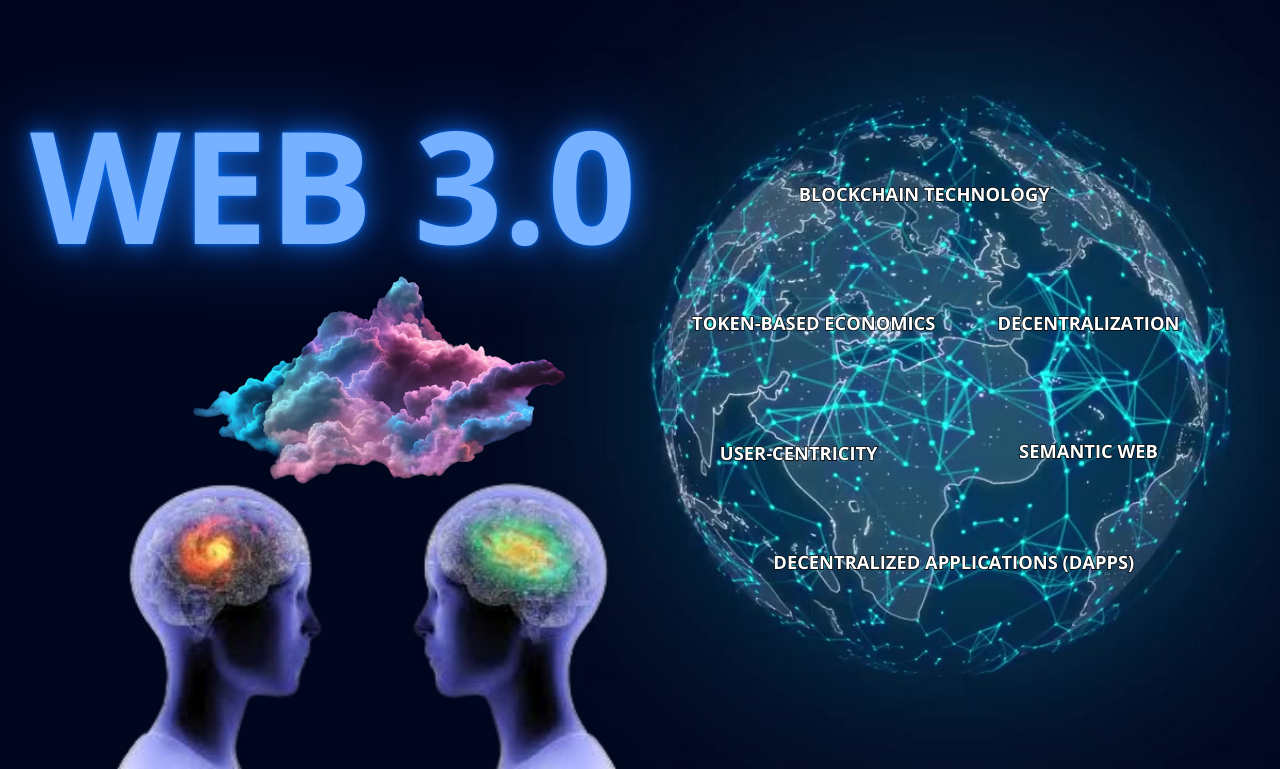
Introduction
The internet has evolved significantly over the years, from Web1 (static pages) to Web2 (interactive, user-generated content) and now Web3, which promises a decentralized, user-controlled internet powered by blockchain technology. At the core of Web3 development is Web3.js, a JavaScript library that allows developers to interact with the Ethereum blockchain.
In this article, we will explore the concept of Web3, the role of Web3.js, its features, and how to use it in real-world applications.
Understanding Web3
Web3 is the next iteration of the internet, characterized by decentralization, blockchain integration, and smart contract functionality. Unlike Web2, where centralized entities control data and services, Web3 aims to distribute power to users via blockchain technology.
Key Features of Web3:
- Decentralization: Shifts control from centralized entities to users, distributing power across networks.
- Blockchain Technology: Ensures secure, transparent transactions and data storage.
- AI Integration: Enhances personalization, smart applications, and data analysis.
- Semantic Web: Enables machines to understand and interpret content for smarter interactions.
- User Ownership: Grants individuals control over their data and digital assets.
- Decentralized Applications (dApps): Run on blockchain networks, offering innovative solutions.
- Smart Contracts: Self-executing agreements ensuring trustless automation.
- Data Ownership: Empowers users with control over personal information.
- Trustless & Permissionless Systems: Eliminates intermediaries, enabling open interactions.
- 3D & Spatial UX: Supports immersive digital experiences.
- Metaverse Support: Forms the foundation for virtual worlds and interactions.
What is Web3.js?
Web3.js is a JavaScript library developed by the Ethereum Foundation that allows developers to interact with the Ethereum blockchain. It provides functionalities such as sending transactions, reading blockchain data, and deploying smart contracts.
Why Use Web3.js?
- Interacting with Smart Contracts: Web3.js enables users to call smart contract functions directly from their applications.
- Managing Ethereum Accounts: It allows users to connect their wallets like MetaMask for seamless transactions.
- Blockchain Data Retrieval: Developers can fetch real-time data from the Ethereum blockchain.
- Transaction Processing: Enables users to send and receive ETH and ERC-20 tokens.
Installing Web3.js
To use Web3.js, you need to install it in your JavaScript project. You can do this using npm or yarn:
npm install web3
or
yarn add web3
After installation, you can import it into your project:
const Web3 = require('web3');
If using ES6 modules:
import Web3 from 'web3';
Connecting to the Ethereum Network
To interact with Ethereum, you need to connect Web3.js to a provider. The most commonly used provider is Infura, but you can also use Alchemy, MetaMask, or your own Ethereum node.
Example using Infura:
const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'));
Example using MetaMask:
const web3 = new Web3(window.ethereum);
await window.ethereum.request({ method: 'eth_requestAccounts' });
Working with Ethereum Accounts
Creating an Ethereum Account
You can create an Ethereum account programmatically using Web3.js:
const account = web3.eth.accounts.create();
console.log(account);
Getting the Balance of an Address
To fetch the balance of an Ethereum address:
const address = '0xYourEthereumAddress';
web3.eth.getBalance(address).then(balance => {
console.log(web3.utils.fromWei(balance, 'ether'), 'ETH');
});
Sending Transactions
Sending Ether
You can send ETH from one account to another using Web3.js:
const transaction = {
from: '0xSenderAddress',
to: '0xReceiverAddress',
value: web3.utils.toWei('0.1', 'ether'),
gas: 21000
};
web3.eth.sendTransaction(transaction)
.on('receipt', console.log);
Interacting with Smart Contracts
To interact with a deployed smart contract, you need its ABI (Application Binary Interface) and contract address.
Example: Reading Data from a Smart Contract
const contractABI = [ /* ABI JSON here */ ];
const contractAddress = '0xYourContractAddress';
const contract = new web3.eth.Contract(contractABI, contractAddress);
contract.methods.getData().call().then(console.log);
Example: Writing Data to a Smart Contract
contract.methods.setData('New Data').send({ from: '0xYourEthereumAddress' });
Using Web3.js with Frontend Applications
Many dApps use Web3.js to connect with Ethereum wallets like MetaMask. Here’s a simple example:
if (window.ethereum) {
window.web3 = new Web3(window.ethereum);
window.ethereum.request({ method: 'eth_requestAccounts' })
.then(accounts => console.log('Connected account:', accounts[0]))
.catch(err => console.error(err));
} else {
console.log('Please install MetaMask');
}
Advanced Web3.js Features
Event Listening
Smart contracts emit events when specific actions occur. You can listen for these events using Web3.js:
contract.events.Transfer({ filter: { from: '0xSenderAddress' } }, (error, event) => {
console.log(event);
});
Gas Fee Estimation
Before sending a transaction, you can estimate the required gas fees:
contract.methods.setData('New Data').estimateGas({ from: '0xYourAddress' })
.then(gas => console.log('Estimated Gas:', gas));
Alternatives to Web3.js
While Web3.js is widely used, other libraries also facilitate Ethereum development:
- Ethers.js: Lightweight and developer-friendly alternative to Web3.js.
- Vieme: Modern TypeScript-based Ethereum library.
- Hardhat & Truffle: Development frameworks for testing and deploying smart contracts.
Final thought
Web3.js is an essential tool for Ethereum blockchain development. It enables interaction with smart contracts, transaction processing, and integration with decentralized applications. By understanding its functionalities, developers can build robust Web3 applications.
Whether you’re working on a decentralized finance (DeFi) project, an NFT marketplace, or a blockchain-based voting system, Web3.js provides the necessary tools to make your vision a reality.