Understanding useState in React: A Complete Guide
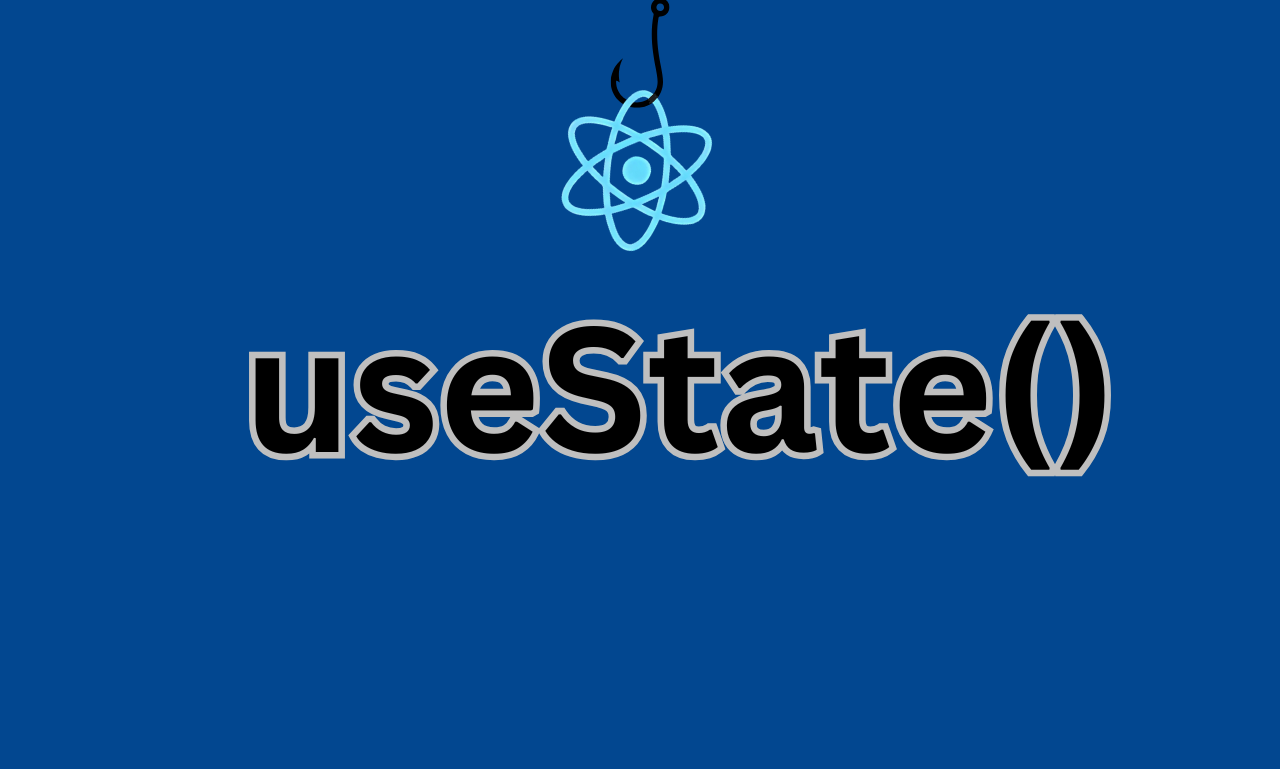
Introduction
React is a powerful JavaScript library for building user interfaces, and one of its key features is the ability to manage component state efficiently. One of the most commonly used React hooks for state management is useState. This hook allows developers to add state to functional components, enabling them to handle dynamic data updates without needing class components. In this article, we will explore useState in depth, understand how it works, and examine real-world use cases.
What is useState?
useState is a built-in React hook that enables functional components to have state variables. In traditional React class components, state was managed using the this.state object. However, with the introduction of hooks in React 16.8, useState allowed functional components to manage their own state without converting them into class components.
The useState hook takes an initial state as an argument and returns an array with two elements:
1.The current state value
2. A function to update the state
Syntax of useState
import React, { useState } from 'react';
function ExampleComponent() { const [count, setCount] = useState(0);
return (
<div>
<p>Current Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase Count</button>
</div>
);
}
export default ExampleComponent;
Explanation:
- useState(0) : Initializes count with 0.
- count : Stores the current state value.
- setCount : A function to update the state.
- onClick={() => setCount(count + 1)} : Updates count when the button is clicked.
Where to Use useState
1. Managing Form Inputs
function FormComponent(){
const [name, setName] = useState('');
return (
<form>
<input type="text" value={name} onChange={(e) =>
setName(e.target.value)} />
<p>Your name: {name}</p>
</form>
);
}
2. Toggling UI Elements
function ToggleComponent() {
const [isVisible, setIsVisible] = useState(false);
return (
<div>
<button onClick={() => setIsVisible(!isVisible)}>Toggle</button> {isVisible && <p>This text appears and disappears.</p>}
</div>
);
}
3. Handling Arrays with useState
function ListComponent() {
const [items, setItems] = useState([]);
const addItem = () => {
setItems([...items, `Item ${items.length + 1}`]);
};
return (
<div>
<button onClick={addItem}>Add Item</button>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
4. Using Objects as State
function ObjectStateComponent() {
const [user, setUser] = useState({ name: '', age: '' });
return (
<div>
<input type="text" placeholder="Name" onChange={(e) =>
setUser({...user, name: e.target.value })} />
<input type="number" placeholder="Age" onChange={(e) =>
setUser({...user, age: e.target.value })} />
<p>Name: {user.name}, Age: {user.age}</p>
</div>
);
}
Best Practices for Using useState
1. Use Functional Updates for Dependent State Changes
When updating state based on the previous state, use the function form:
setCount(prevCount => prevCount + 1);
2. Avoid Unnecessary Re-renders
Using multiple state variables instead of one large object can help prevent unnecessary re-renders.
3. Initialize State Correctly
If the initial state requires complex calculations, use a function:
const [data, setData] = useState(() => expensiveCalculation());
4. Use State Immutability
Always create a new state object instead of modifying the existing one directly.
Common Mistakes with useState
1. Modifying State Directly
Incorrect:
user.name = 'John'; // This does not trigger a re-render
Correct:
setUser({ ...user, name: 'John' });
2. Using State Incorrectly in Event Handlers
Incorrect:
setCount(count + 1);setCount(count + 1); // Will not work as expected
Correct:
setCount(prevCount => prevCount + 1);
setCount(prevCount => prevCount + 1); // Ensures correct updates
3. Not Using useEffect for Side Effects
If state updates require API calls or local storage updates, use useEffect.
useEffect(() => { localStorage.setItem(‘count’, count);}, [count]);
Final thought
useState
is an essential React hook for managing component state in functional components. It simplifies state handling, makes components more readable, and eliminates the need for class-based state management. By understanding its proper usage and avoiding common mistakes, developers can build more efficient and responsive React applications.
By following best practices and real-world use cases, you can harness the full potential of useState
to build dynamic, interactive web applications. Happy coding!